· tutorials · 4 min read
How To Convert HTML to PDF with PHP
Master the art of converting HTML to PDF in PHP by exploring the most effective libraries and tools.
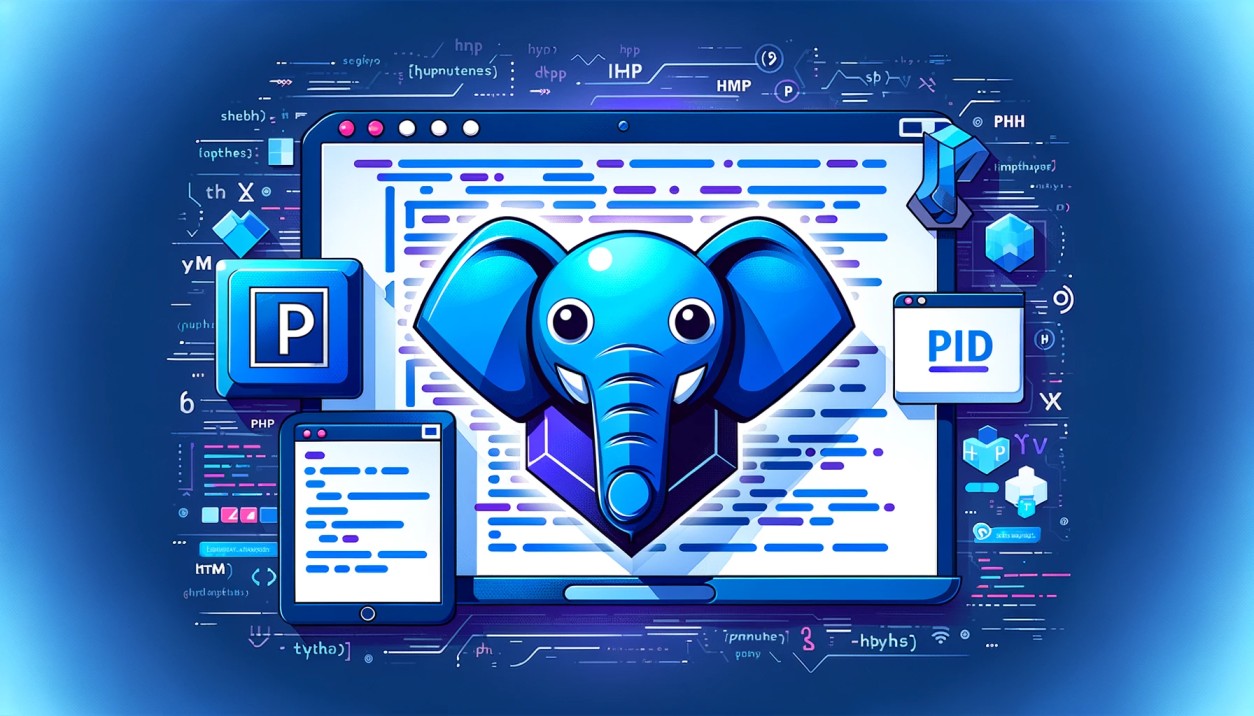
Introduction
Converting HTML to PDF is a frequent requirement for PHP developers, especially when dealing with report generation, invoice creation, or simply rendering user-generated content for offline use. In this article, we explore various PHP libraries that facilitate this conversion, guiding you through a step-by-step approach to select and use the most efficient tool for your project needs.
Dompdf: A PHP Library
Dompdf is a popular PHP library that offers a straightforward way to convert HTML to PDF. It supports CSS styles, which means you can style your HTML as needed before converting it.
Step 1: Install Dompdf
Use Composer to install Dompdf in your project:
composer require dompdf/dompdf
Step 2: Convert HTML to PDF
<?phprequire 'vendor/autoload.php';use Dompdf\Dompdf;
$dompdf = new Dompdf();$dompdf->loadHtml('<h1>Hello, Dompdf!</h1>');
$dompdf->setPaper('A4', 'landscape');$dompdf->render();$dompdf->stream("sample.pdf");?>
This code initializes Dompdf, loads HTML content, sets the paper size, renders the PDF, and streams it to the browser.
TCPDF: Advanced PDF Generation
For more complex requirements, TCPDF is a powerful library that supports a wide range of PDF features.
Step 1: Install TCPDF
composer require tecnickcom/tcpdf
Step 2: Generate PDF with TCPDF
<?phprequire_once('vendor/autoload.php');$pdf = new TCPDF();
$pdf->AddPage();$pdf->SetFont('helvetica', '', 12);$pdf->Write(0, 'Hello, TCPDF!', '', 0, 'L', true, 0, false, false, 0);
$pdf->Output('example.pdf', 'I');?>
This snippet creates a new PDF, adds a page, sets the font, writes text to the page, and outputs the PDF to the browser.
Comparison Table
Feature | Dompdf | TCPDF |
---|---|---|
Ease of Use | Easy for basic needs | Moderate for advanced features |
CSS Support | Yes, with limitations | Extensive, with CSS and HTML5 support |
Performance | Good for small to medium-sized documents | Optimized for larger documents |
Customization | Limited compared to TCPDF | Highly customizable |
Both Dompdf and TCPDF offer robust solutions for converting HTML to PDF in PHP. Your choice depends on the complexity of your documents and the level of customization required. For simple projects, Dompdf might be the go-to choice for its ease of use, while TCPDF is better suited for more complex documents that require fine-grained control over the PDF generation process.
A Better Approach: HTML to PDF using Templated
For tasks like generating PDFs from templates or managing generated PDFs, Templated offers a powerful and efficient solution. Templated’s API simplifies the process, eliminating the need for extensive coding or manual template management.
Using Templated you can design your PDF template using a drag-and-drop editor.
Check the video below to see an example of the editor:
Using Templated with PHP
Step 1: Set Up Templated API
Templated’s API can be easily integrated into your PHP application, allowing you to leverage its capabilities for PDF generation with minimal effort. After logging in you will see your Dashboard where you can manage your templated or create new ones:
Ensure you have an account on Templated and obtain your API key from the dashboard. Use curl
or any HTTP client in PHP to make API requests.
Step 2: Generate PDF with Templated
Here’s a PHP example using curl
to make a POST request to Templated’s API:
<?php$curl = curl_init();
curl_setopt_array($curl, array( CURLOPT_URL => 'https://api.templated.io/v1/render', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => json_encode(array( "template" => "your-template-id", "format" => "pdf", "layers" => array( "date" => array("text" => "02/10/2024"), "name" => array("text" => "John Doe"), "signature" => array("text" => "Dr. Mark Brown"), "details" => array("text" => "This certificate is awarded to John Doe in recognition of their successful completion of Computer Science Degree on 02/10/2024.") ) )), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer API_KEY', // Replace YOUR_API_KEY with your actual API key 'Content-Type: application/json' ),));
$response = curl_exec($curl);
curl_close($curl);echo $response;?>
Replace your-template-id
with your template ID and API_KEY
with your actual API key from Templated.
Conclusion
While PHP offers libraries like Dompdf and TCPDF for HTML to PDF conversion, Templated provides a more streamlined and scalable solution. With Templated, you can focus on your application’s core features, leaving the complexities of PDF generation to a robust and reliable platform.