· tutorials · 3 min read
How To Convert HTML to PDF with Node.js
Explore step-by-step methods to convert HTML to PDF in Node.js and compare the top libraries to find the best fit for your project.
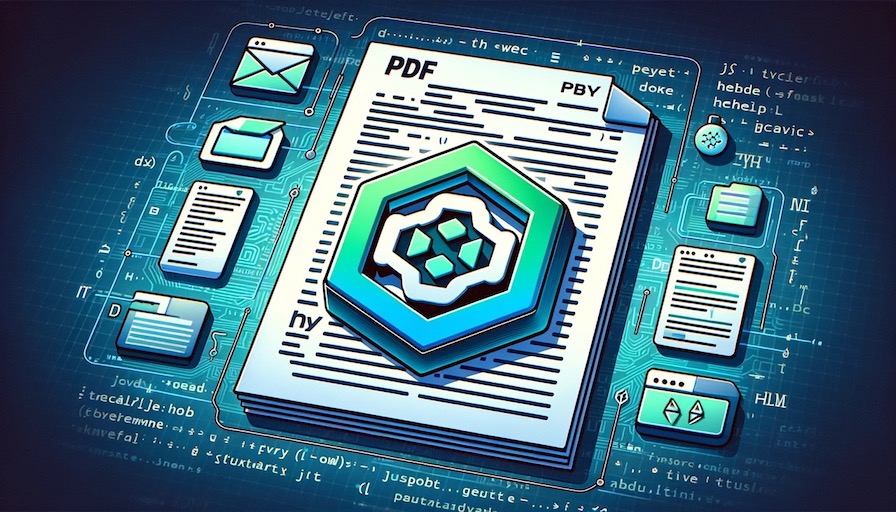
Introduction
This guide provides a step-by-step approach to converting HTML to PDF using Node.js, covering popular libraries like Puppeteer, jsPDF, and html-pdf. We’ll conclude with a comparison table to help you select the right tool for your needs.
Puppeteer: Full Web Page Rendering
Step 1: Install Puppeteer
npm install puppeteer
Step 2: Convert HTML to PDF
const puppeteer = require('puppeteer');
async function createPDF() { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.setContent('<h1>Hello, Puppeteer!</h1>'); await page.pdf({ path: 'example.pdf', format: 'A4' }); await browser.close();}
createPDF();
jsPDF: Client-Side PDF Generation
Step 1: Include jsPDF
In Node.js, you can install jsPDF using npm:
npm install jspdf
Step 2: Generate PDF
const { jsPDF } = require("jspdf");const doc = new jsPDF();
doc.text("Hello World!", 10, 10);doc.save("a4.pdf");
html-pdf: Simple HTML to PDF Conversion
Step 1: Install html-pdf
npm install html-pdf
Step 2: Create PDF from HTML
const pdf = require('html-pdf');const html = '<b>Hello world</b>';
pdf.create(html, { format: 'Letter' }).toFile('./output.pdf', (err, res) => { if (err) return console.log(err); console.log(res);});
Comparison Table
Feature | Puppeteer | jsPDF | html-pdf |
---|---|---|---|
Execution | Server-side (Node) | Client/Server-side | Server-side (Node) |
Browser Engine | Chromium | N/A | PhantomJS |
JavaScript Support | Yes | Limited | No |
CSS Support | Full | Limited | Basic |
API Complexity | Moderate | Simple | Simple |
Use Case | Complex web pages | Simple documents | Basic HTML content |
Templated: A template-based solution
For those looking for a more streamlined approach, especially when dealing with dynamic content or templates, Templated offers an efficient solution. Templated simplifies the process of generating PDFs from HTML content using an API-based platform, powered by a Chromium-based rendering engine that fully supports JavaScript, CSS, and HTML.
With Templated you can design your PDF template using a drag-and-drop editor and then create PDFs using an API.
Check the video below to see an example of the editor:
Step 1: Set Up Templated API
First, ensure you have an account with Templated and obtain your API key from the dashboard. After logging in you will see your Dashboard where you can manage your templates or create new ones:
Then, set up your Node.js project to make HTTP requests. You can use libraries like axios
for this purpose.
npm install axios
Step 2: Generate PDF with Templated
Create a Node.js script to call the Templated API, passing in your template data and desired options. Here’s an example of generating a PDF using Templated:
const axios = require('axios');
async function createPDFwithTemplated() { try { const payload = { template: "template_id", format: "pdf", layers: { date: { text: "02/10/2024" }, name: { text: "John Doe" }, signature: { text: "Dr. Mark Brown" }, details: { text: "This certificate is awarded to John Doe in recognition of their successful completion of Computer Science Degree on 02/10/2024." } } };
const response = await axios.post('https://api.templated.io/v1/render', payload, { headers: { 'Content-Type': 'application/json', 'Authorization': 'Bearer ${YOUR_API_KEY}' // Replace ${YOUR_API_KEY} with your actual API key } });
console.log("Response Code:", response.status); console.log(response.data); // The API response } catch (error) { console.error(error); }}
createPDFwithTemplated();
In this example, replace template_id
with the actual ID of your template and YOUR_API_KEY
with your Templated API key. The data
object should contain the data you want to use in your template.
Other languages
You you want to learn how to convert HTML to PDF in other languages here are other resources for you to explore:
- How To Convert HTML to PDF with Java
- How To Convert HTML to PDF with C#
- How To Convert HTML to PDF with PHP
- How To Convert HTML to PDF with Python
Conclusion
Templated provides a powerful yet simple solution for generating PDFs, especially useful for applications requiring dynamic content generation, template management, and tracking. By leveraging Templated’s API, you can focus on your application’s core functionality without worrying about the complexities of PDF generation.
Automate your content with Templated