· tutorials · 3 min read
How To Convert HTML to PDF with C#
Discover efficient techniques to convert HTML to PDF in C# applications, including a superior solution with Templated.
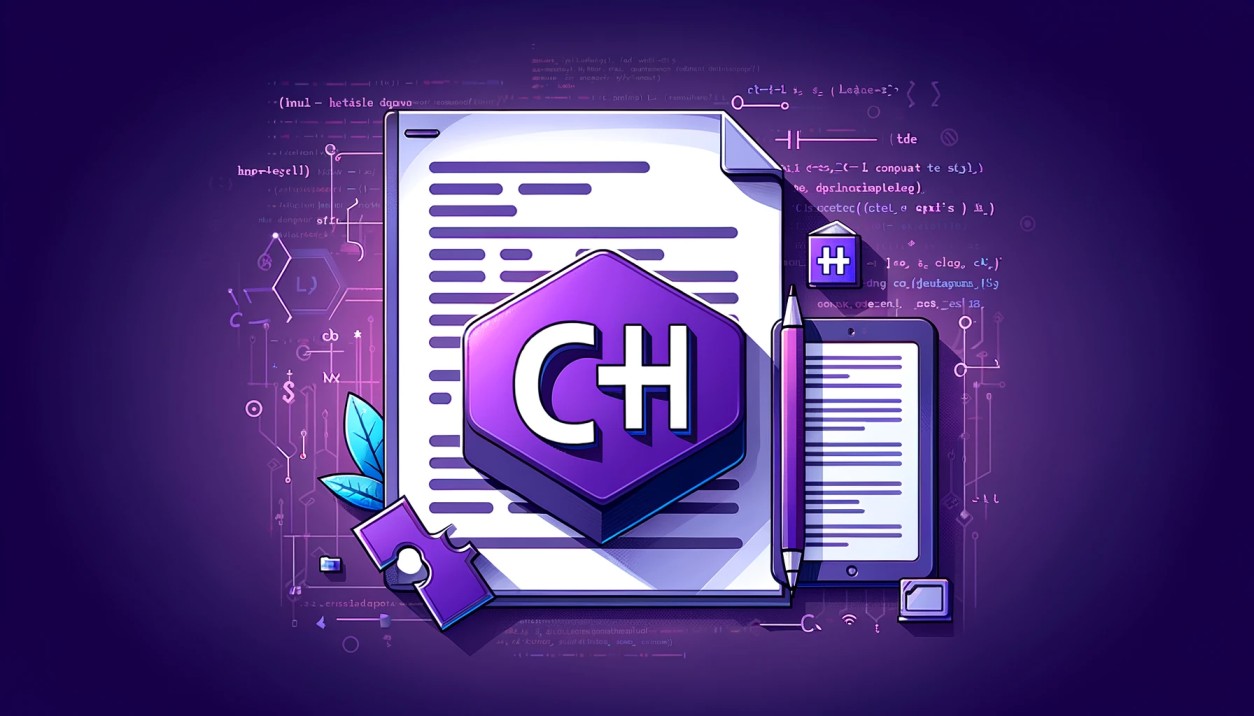
Introduction
In C# development, converting HTML to PDF is a common task, often required in web and desktop applications for report generation, document archiving, and more. This article explores various libraries available for C# and introduces Templated as an advanced solution for managing templates and generating PDFs efficiently.
iTextSharp: A Popular C# Library
iTextSharp is widely used for PDF manipulation in C#, including HTML to PDF conversion.
Step 1: Install iTextSharp
Add iTextSharp to your project via NuGet Package Manager:
Install-Package itext7
Step 2: Convert HTML to PDF with iTextSharp
using iText.Html2pdf;
public class HtmlToPdfConverter { public void ConvertHtmlToPdf(string html, string outputPath) { HtmlConverter.ConvertToPdf(html, new FileStream(outputPath, FileMode.Create)); }}
This code snippet demonstrates how to use iTextSharp to convert an HTML string to a PDF file.
Templated: Streamlining PDF Generation
While libraries like iTextSharp provide the tools for HTML to PDF conversion, Templated offers a more streamlined and scalable approach, especially for applications requiring dynamic content generation and template management.
Using Templated you can design your PDF template using a drag-and-drop editor.
Check the video below to see an example of the editor:
Using Templated with C#
Step 1: Set Up Templated API
Templated’s API can be easily integrated into your PHP application, allowing you to leverage its capabilities for PDF generation with minimal effort. After logging in you will see your Dashboard where you can manage your templated or create new ones:
Integrate Templated’s API into your C# application to leverage powerful PDF generation capabilities with minimal effort.
Ensure you have a Templated account and your API key. Use HttpClient or any other HTTP client in C# to make API requests.
Step 2: Generate PDF with Templated
Here’s a C# example using HttpClient to make a POST request to Templated’s API:
using System.Net.Http;using System.Text;using System.Threading.Tasks;
public class TemplatedApiClient { public async Task GeneratePdf() { var client = new HttpClient(); var payload = new StringContent(@"{ ""template"": ""your-template-id"", ""data"": { ""date"": ""02/10/2024"", ""name"": ""John Doe"", ""signature"": ""Dr. Mark Brown"", ""details"": ""This certificate is awarded to John Doe..."" } }", Encoding.UTF8, "application/json");
client.DefaultRequestHeaders.Add("Authorization", "Bearer YOUR_API_KEY"); var response = await client.PostAsync("https://api.templated.io/pdf/generate", payload); var responseString = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseString); }}
Replace 'your-template-id'
and 'YOUR_API_KEY'
with your actual template ID and API key from Templated.
Conclusion
C# developers have multiple options for converting HTML to PDF, but Templated stands out for its ease of use, especially when dealing with dynamic content and templates. By integrating Templated into your application, you can significantly simplify PDF generation and focus on your application’s core functionality.