· tutorials · 6 min read
How To Convert HTML to PDF with C#
Discover efficient techniques to convert HTML to PDF in C# applications, including popular libraries and a better approach.
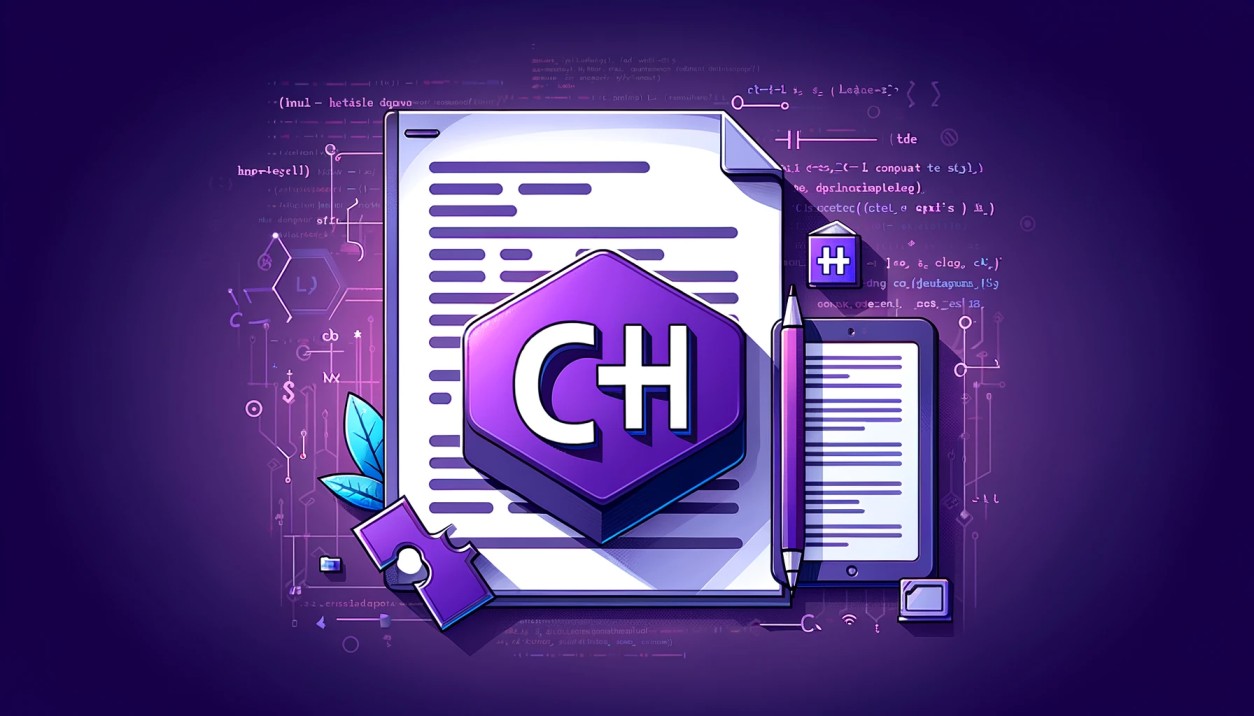
Introduction
Converting HTML to PDF is a common requirement in C# development, often needed for report generation, document archiving, and creating printable versions of web content. In 2024, C# developers have access to various tools and libraries that simplify this process. This article explores some of the most efficient methods for converting HTML to PDF using C#, including popular libraries and advanced solutions.
Why Convert HTML to PDF?
- Consistent Formatting: PDFs maintain their layout across different devices and platforms.
- Document Integrity: PDFs are less easily altered than HTML pages, preserving document integrity.
- Offline Access: PDFs can be easily downloaded and viewed offline.
- Print-Friendly: PDFs are optimized for printing, maintaining the intended layout.
- Universal Compatibility: PDF is a widely accepted format in business and legal contexts.
HTML to PDF Conversion Using C# Libraries
1. iTextSharp
iTextSharp is a popular open-source library for PDF generation and manipulation in C#.
Step 1: Install iTextSharp
Add iTextSharp to your project via NuGet Package Manager:
Install-Package itext7
Step 2: Convert HTML to PDF with iTextSharp
Here’s a basic C# code snippet demonstrating HTML to PDF conversion using iTextSharp:
using iText.Html2pdf;using iText.Kernel.Pdf;using System.IO;
public class HtmlToPdfConverter{ public void ConvertHtmlToPdf(string html, string outputPath) { using (FileStream fs = new FileStream(outputPath, FileMode.Create)) { ConverterProperties converterProperties = new ConverterProperties(); HtmlConverter.ConvertToPdf(html, fs, converterProperties); } Console.WriteLine("PDF created successfully at: " + outputPath); }}
// Usagevar converter = new HtmlToPdfConverter();string html = "<html><body><h1>Hello, iTextSharp!</h1></body></html>";converter.ConvertHtmlToPdf(html, "output.pdf");
This code demonstrates a simple conversion of HTML content to a PDF document using iTextSharp.
2. PdfSharp
PdfSharp is another popular library for creating and processing PDF documents in C#.
Step 1: Install PdfSharp
Add PdfSharp to your project via NuGet Package Manager:
Install-Package PdfSharp
Step 2: Convert HTML to PDF with PdfSharp
Here’s a basic example of using PdfSharp to create a PDF (note that PdfSharp doesn’t directly convert HTML, but can be used to create PDFs programmatically):
using PdfSharp.Pdf;using PdfSharp.Drawing;
public class PdfCreator{ public void CreatePdf(string outputPath) { PdfDocument document = new PdfDocument(); PdfPage page = document.AddPage(); XGraphics gfx = XGraphics.FromPdfPage(page); XFont font = new XFont("Verdana", 20, XFontStyle.Bold);
gfx.DrawString("Hello, PdfSharp!", font, XBrushes.Black, new XRect(0, 0, page.Width, page.Height), XStringFormats.Center);
document.Save(outputPath); Console.WriteLine("PDF created successfully at: " + outputPath); }}
// Usagevar creator = new PdfCreator();creator.CreatePdf("output.pdf");
3. Syncfusion PDF Library
Syncfusion offers a comprehensive PDF library for C# that includes HTML to PDF conversion capabilities.
Step 1: Install Syncfusion PDF Library
Add the Syncfusion PDF library to your project via NuGet Package Manager:
Install-Package Syncfusion.Pdf.Net.CoreInstall-Package Syncfusion.HtmlToPdfConverter.Net.Core
Step 2: Convert HTML to PDF with Syncfusion
Here’s an example of using Syncfusion to convert HTML to PDF:
using Syncfusion.Pdf;using Syncfusion.HtmlConverter;
public class SyncfusionHtmlToPdfConverter{ public void ConvertHtmlToPdf(string html, string outputPath) { HtmlToPdfConverter htmlConverter = new HtmlToPdfConverter(); PdfDocument document = htmlConverter.Convert(html); document.Save(outputPath); document.Close(true); Console.WriteLine("PDF created successfully at: " + outputPath); }}
// Usagevar converter = new SyncfusionHtmlToPdfConverter();string html = "<html><body><h1>Hello, Syncfusion!</h1></body></html>";converter.ConvertHtmlToPdf(html, "output.pdf");
Comparing the Libraries
When selecting a C# library for HTML to PDF conversion, consider factors such as features, ease of use, and specific project requirements. Here’s a comparison table:
Library | Pros | Cons | Best For |
---|---|---|---|
iTextSharp | Powerful and flexible. Good for complex PDF tasks. | Steep learning curve. Community version has limitations. | Advanced PDF manipulation and generation. |
PdfSharp | Lightweight and easy to use. Open-source. | Limited HTML to PDF conversion capabilities. | Simple PDF creation and manipulation tasks. |
Syncfusion | Comprehensive features. Good documentation. | Commercial license required. | Enterprise-level applications with diverse PDF needs. |
Simplifying HTML to PDF Conversion with Templated
While the above libraries offer powerful capabilities, they often require significant setup and maintenance. Templated provides a more streamlined approach, especially for applications that need dynamic content generation and template management.
Traditional libraries often struggle with template management, PDF tracking, and complex rendering. Templated solves these issues by offering:
- A drag-and-drop template editor
- Automatic PDF tracking
- Chromium-based rendering for accurate results
- A scalable API approach
With Templated, you can streamline PDF creation, saving time and ensuring high-quality output.
This approach simplifies the process and eliminates the need for extensive manual template management and tracking. To get started with PDF generation using Templated, follow the documentation and harness the power of this efficient solution.
1. Create PDFs with templates
Using Templated, you can design your PDF template using a drag-and-drop editor and then generate your PDFs with an API. Check the video below to see an example of the editor:
After logging in, you will see your Dashboard where you can manage your templates or create new ones:
From your Dashboard, you can design your own templates or customize an existing one from our Template Gallery. Below is the Certificate of Achievement Template you can use. There are 100+ free templates available that you can pick and customize to your needs.
To start using Templated API, you need to get your API key that can be found on the API Integration tab on your dashboard.
Using Templated with C#
Now that you have your Templated account ready, let’s see how you can integrate your application with the API. In this example, we’ll be using a certificate template to generate PDFs.
Here’s an updated C# example using HttpClient to make a POST request to Templated’s API:
using System;using System.Net.Http;using System.Text;using System.Threading.Tasks;using Newtonsoft.Json;
public class TemplatedApiClient{ private readonly HttpClient _client = new HttpClient(); private const string ApiUrl = "https://api.templated.io/v1/render";
public TemplatedApiClient(string apiKey) { _client.DefaultRequestHeaders.Add("Authorization", $"Bearer {apiKey}"); }
public async Task GeneratePdf() { var payload = new { template = "your-template-id", format = "pdf", layers = new { date = new { text = "02/10/2024" }, name = new { text = "John Doe" }, signature = new { text = "Dr. Mark Brown" }, details = new { text = "This certificate is awarded to John Doe..." } } };
var content = new StringContent(JsonConvert.SerializeObject(payload), Encoding.UTF8, "application/json");
var response = await _client.PostAsync(ApiUrl, content); var responseString = await response.Content.ReadAsStringAsync();
Console.WriteLine(responseString); }}
// Usagevar client = new TemplatedApiClient("YOUR_API_KEY");await client.GeneratePdf();
Replace 'your-template-id'
and 'YOUR_API_KEY'
with your actual template ID and API key from Templated.
When you run this code, you’ll receive a response similar to:
{"renderUrl":"PDF_URL","status":"success","template":"YOUR_TEMPLATE_ID"}
In this example, using Templated to convert HTML to PDF is straightforward. No additional libraries need to be installed. You only need to make a single API call, providing your data as the request body. That’s all there is to it!
You can use the renderUrl
from the response to download or distribute the generated PDF.
Conclusion
C# developers have multiple options for converting HTML to PDF, each with its own strengths and use cases. While libraries like iTextSharp, PdfSharp, and Syncfusion offer powerful features, they often require significant setup and maintenance.
Templated stands out for its ease of use, especially when dealing with dynamic content and templates. By integrating Templated into your application, you can significantly simplify PDF generation and focus on your application’s core functionality.
For more information on HTML to PDF conversion in other languages, check out our guides for Python, Java, PHP, and Node.js.
To get started with Templated and streamline your PDF generation process, sign up for a free account today!
Automate your content with Templated