· tutorials · 5 min read
How to Convert HTML to PDF using OpenPDF
Learn the step-by-step process to efficiently convert HTML to PDF in Java applications using the OpenPDF library
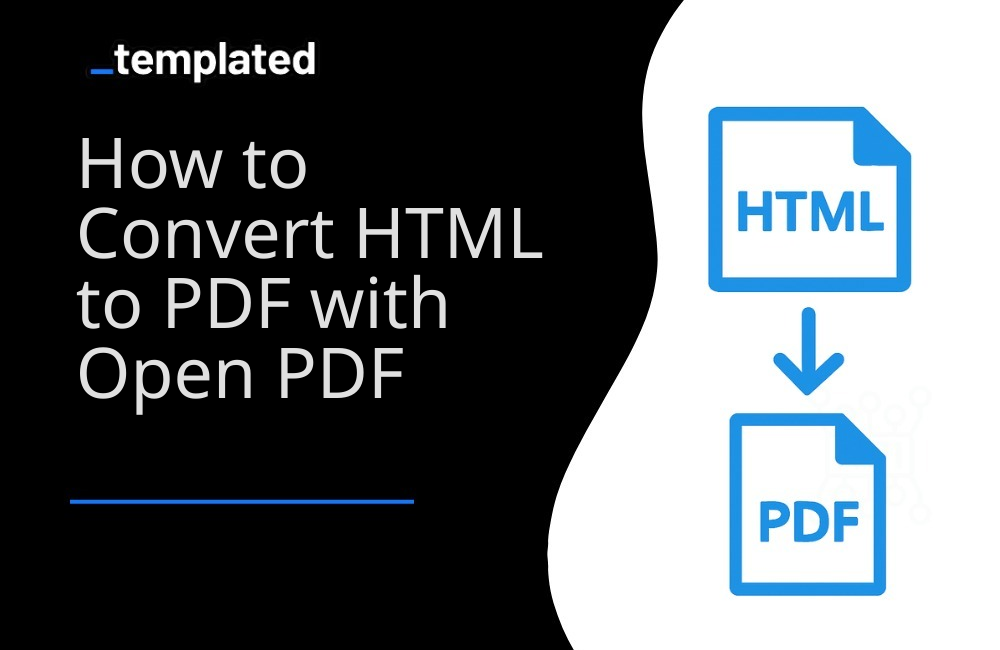
Introduction
In the realm of Java development, generating PDF documents from HTML content is a frequent requirement, especially in web and enterprise applications. OpenPDF, a free and open-source Java library, stands out for this task due to its ease of use and efficiency. This article provides a comprehensive guide on converting HTML to PDF using OpenPDF in Java, complete with code examples to get you started.
Why Choose OpenPDF?
- Open Source: OpenPDF is entirely open-source, allowing for customization and community-driven enhancements.
- Simplicity: It offers a straightforward API, making it accessible for beginners and efficient for experienced developers.
- Compatibility: OpenPDF is compatible with a wide range of Java applications, ensuring seamless integration.
- Feature-Rich: Despite its simplicity, OpenPDF supports a variety of PDF features, including document manipulation, text formatting, and image inclusion.
Getting Started with OpenPDF
Step 1: Add OpenPDF Dependency
To begin, you need to include OpenPDF in your Java project. For Maven users, add the following dependency to your pom.xml
file:
xmlCopy code
<dependency> <groupId>com.github.librepdf</groupId> <artifactId>openpdf</artifactId> <version>1.3.26</version></dependency>
This dependency will include OpenPDF in your project, enabling you to use its functionality to create and manipulate PDF documents.
Step 2: Convert HTML to PDF
Once OpenPDF is added to your project, you can start converting HTML content to PDF. The following Java code example illustrates this process:
import com.lowagie.text.Document;import com.lowagie.text.Paragraph;import com.lowagie.text.html.simpleparser.HTMLWorker;import com.lowagie.text.pdf.PdfWriter;import java.io.FileOutputStream;import java.io.StringReader;
public class HtmlToPdfOpenPDF { public static void main(String[] args) { Document document = new Document(); try { PdfWriter.getInstance(document, new FileOutputStream("ConvertedHtmlToPdf.pdf")); document.open(); String htmlContent = "<html><head><title>Sample HTML</title></head><body><h1>Hello, OpenPDF!</h1><p>This is a paragraph in the generated PDF.</p></body></html>"; HTMLWorker htmlWorker = new HTMLWorker(document); htmlWorker.parse(new StringReader(htmlContent)); } catch (Exception e) { e.printStackTrace(); } finally { document.close(); } System.out.println("PDF created successfully."); }}
In this example:
- A
Document
object is created, representing the PDF document. - The
PdfWriter
instance links the document to a file output stream, specifying the PDF file’s name. - The
HTMLWorker
class parses the HTML content and adds it to theDocument
. - Finally, the document is closed, and the PDF is saved with the HTML content rendered inside.
Advanced Features
While the above example covers basic HTML to PDF conversion, OpenPDF also supports more advanced features, such as:
- Styling with CSS: You can include CSS styles within your HTML content to control the appearance of the PDF content.
- Image Inclusion: HTML tags for images (
<img>
) are supported, allowing you to embed images in your PDF. - Font Customization: OpenPDF allows for font customization, enabling you to maintain brand consistency or enhance the document’s aesthetics.
Adding CSS and Images
To include CSS and images in your HTML content, ensure that your HTML string includes the necessary CSS styles and image tags. OpenPDF will process these elements during the conversion.
Custom Fonts
To use custom fonts, you can use OpenPDF’s font classes to define and set fonts in your document. This requires additional steps to load and set the font for document elements.
Creating PDF from a URL
In some cases, you might want to create a PDF from the content available at a specific URL. Here’s how you can achieve this using OpenPDF:
import com.lowagie.text.Document;import com.lowagie.text.html.simpleparser.HTMLWorker;import com.lowagie.text.pdf.PdfWriter;import java.io.BufferedReader;import java.io.FileOutputStream;import java.io.InputStreamReader;import java.net.HttpURLConnection;import java.net.URL;
public class UrlToPdfOpenPDF { public static void main(String[] args) { // Specify the URL of the HTML page String webpageUrl = "http://example.com";
// Initialize a StringBuilder to hold the HTML content StringBuilder htmlStringBuilder = new StringBuilder();
try { // Create a URL object from the webpage URL URL url = new URL(webpageUrl);
// Open a connection to the URL HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// Set the request method to GET connection.setRequestMethod("GET");
// Read the response from the URL BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String inputLine; while ((inputLine = bufferedReader.readLine()) != null) { htmlStringBuilder.append(inputLine); } bufferedReader.close();
// Convert the StringBuilder to a String containing the HTML content String htmlContent = htmlStringBuilder.toString();
// Use OpenPDF to convert the HTML content to a PDF Document document = new Document(); PdfWriter.getInstance(document, new FileOutputStream("UrlToPdf.pdf")); document.open(); HTMLWorker htmlWorker = new HTMLWorker(document); htmlWorker.parse(new InputStreamReader(new java.io.ByteArrayInputStream(htmlContent.getBytes()))); document.close();
System.out.println("PDF created successfully from URL."); } catch (Exception e) { e.printStackTrace(); } }}
This section demonstrates how to programmatically fetch HTML content from a specified URL and convert it into a PDF document using OpenPDF.
Other Java libraries
There are other Java libraries capable of converting HTML to PDF with an API. One popular alternative is Flying Saucer, which excels at converting XHTML/CSS content into PDFs with a high level of precision. You can learn more about using Flying Saucer in our article on How to Convert HTML to PDF using Flying Saucer.
For more information about various Java libraries for HTML to PDF conversion, check out our comprehensive guide on How To Convert HTML to PDF with Java.
Conclusion
OpenPDF provides a robust and straightforward solution for converting HTML to PDF in Java applications. Whether you’re generating reports, invoices, or any other PDF documents from HTML content, OpenPDF offers the tools you need to get the job done efficiently. With its open-source nature and extensive features, OpenPDF is an excellent choice for Java developers looking to integrate HTML to PDF conversion functionality into their applications.
Remember to explore OpenPDF’s documentation and community resources for more advanced use cases and to stay updated with the latest features and best practices. Happy coding!
Automate your content with Templated