· tutorials · 9 min read
How To Convert HTML to PDF with Python
Learn different approaches on how to convert HTML to PDF and pick the one best for your needs
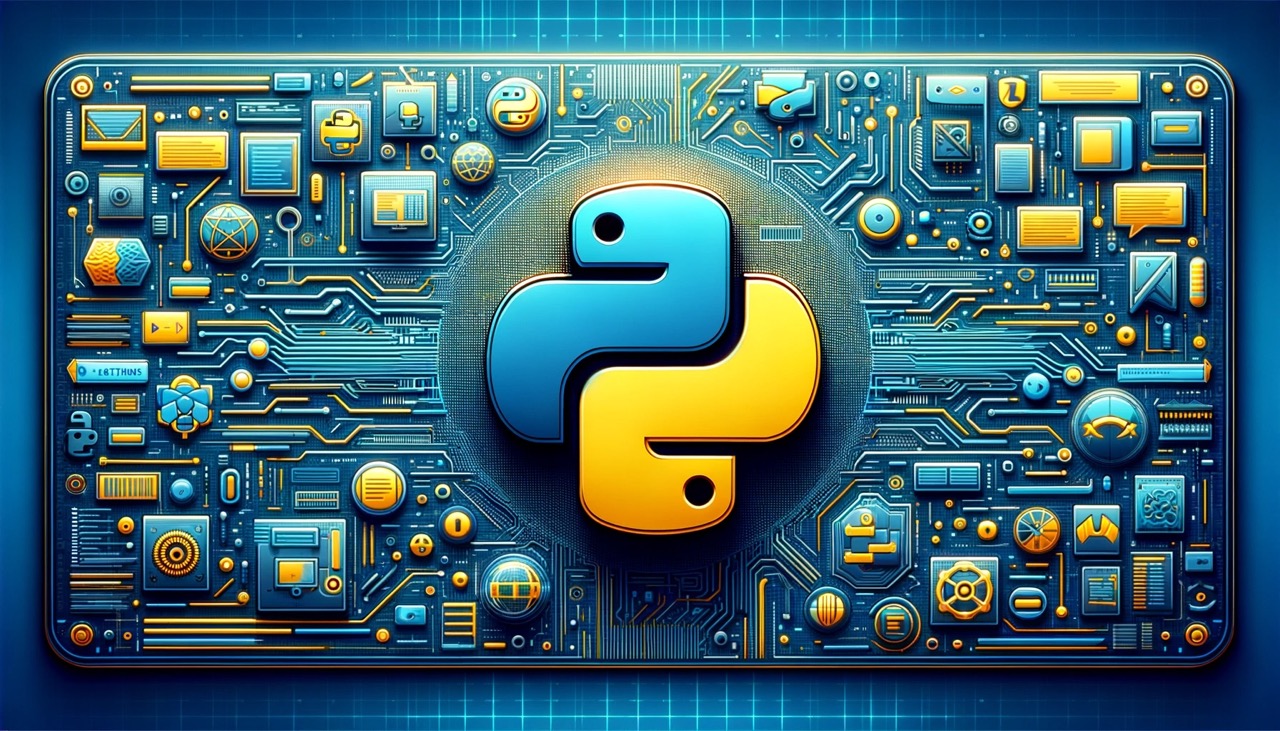
Introduction
Creating PDFs programmatically in Python is a common task, and although there’s no single correct method for doing so, certain approaches can be more efficient and faster than others.
This article explores some of the most efficient methods for converting HTML to PDF using Python.
Why create PDF from HTML?
Here are some reasons on why generating PDFs from HTML is a good idea:
- Market Norm: HTML serves as the internet’s foundation, providing a universally accepted and widespread method for organizing content.
- Established Technology: Open standards ensure HTML compatibility across an extensive array of tools and technologies.
- Flexibility: The availability of numerous tools eases the process of conversion.
- CSS Customization: Employ CSS for intricate styling within PDF documents.
- Media Integration: HTML offers a solid foundation for creating documents enriched with multimedia elements.
To sum up, changing HTML into PDFs brings together the best aspects of both: HTML’s flexibility, accessibility, and interactivity, and the portability and standardization of PDFs.
HTML to PDF using Python Libraries
Many Python libraries exist for creating PDFs from HTML content, and some of these are detailed below.
1. Pyppeteer
Pyppeteer is the Python version of the Node library Puppeteer. It offers a high-level API over the Chrome DevTools Protocol, almost like having a browser in your code that can do things a regular browser does. Puppeteer can be used for tasks like scraping data from websites, taking screenshots, and more. Let’s explore how we can use pyppeteer to create PDFs from HTML..
Step 1: Install Pyppeteer
First, we need to install pyppeteer with the following command:
pip install pyppeteer
Step 2: Python code to convert HTML to PDF with Pyppeteer
import asynciofrom pyppeteer import launch
async def create_pdf(url, pdf_path): browser = await launch() page = await browser.newPage()
await page.goto(url) await page.pdf({'path': pdf_path, 'format': 'A4'}) await browser.close()
# Run create_pdfasyncio.get_event_loop().run_until_complete(generate_pdf('https://google.com', 'google_example.pdf'))
In the generate_pdf
method of the code, the following steps are taken:
- A new browser instance is launched in headless mode.
- A new page is opened in the browser, and it waits until the page is ready.
- The browser navigates to the URL provided in the url argument and waits for the page to fully load.
- A PDF of the webpage is generated and saved to the location specified in pdf_path, with the format (dimensions) set to A4.
- The headless browser is then closed.
Now, to generate a PDF from custom HTML this is the code and process:
import asynciofrom pyppeteer import launch
async def create_pdf_from_html(html, pdf_path): browser = await launch() page = await browser.newPage()
await page.setContent(html) await page.pdf({'path': pdf_path, 'format': 'A4'}) await browser.close()
# HTML contenthtml = '''<html> <head> <title>PDF Example</title> </head>
<body> <h1>Hey, this will turn into a PDF!</h1> </body></html>'''
# Run create_pdf_from_htmlasyncio.get_event_loop().run_until_complete(generate_pdf_from_html(html, 'from_html.pdf'))
The method generate_pdf_from_html
in the Pyppeteer example above demonstrates how to generate PDFs using custom HTML content. Here’s what happens in this approach:
- A new headless browser instance is launched.
- A new page is opened in the headless browser, and it waits until it is ready.
- The content of the page is explicitly set to our custom HTML content.
- A PDF of the webpage is generated and saved to the location specified in pdf_path, with the format set to ‘A4’.
- The headless browser is closed.
2. python-pdfkit
python-pdfkit is a Python wrapper for the wkhtmltopdf utility, which uses Webkit to convert HTML to PDF.
Step 1: Install python-pdfkit
First, let’s install python-pdfkit with pip:
pip install pdfkit
Step 2: Python code to convert website URL to PDF with python-pdfkit
import pdfkit
# URL to fetchurl = 'https://engadget.com'
# PDF path to savepdf_path = 'example.pdf'
pdfkit.from_url(url, pdf_path)
pdfkit supports generating PDFs from website URLs out of the box just like Pyppeteer.
In the above code, as you can see, pdfkit is generating pdf just from one line code. pdfkit.from_url
is all you need to generate a PDF.
Step 3: Generating PDF from custom HTML content
import pdfkit
# HTML contenthtml = '''<html> <head> <title>PDF Example</title> </head>
<body> <h1>Hey, this will turn into a PDF!</h1> </body></html>'''
# PDF path to savepdf_path = 'example.pdf'
# Create PDFpdfkit.from_string(html, pdf_path)
To generate a PDF from custom HTML content using python-pdfkit, you simply need to use pdfkit.from_string
and provide the HTML content along with the path for the PDF file.
3. xhtml2pdf
xhtml2pdf is a Python library that enables the creation of PDFs from HTML content with a slighly different approach. Let’s take a look at xhtml2pdf in use.
Step 1: Install xhtml2pdf
To install xhtml2pdf, use the following command:
pip install xhtml2pdf requests
Step 2: Generate PDF from a website URL with xhtml2pdf:
It’s important to note that xhtml2pdf doesn’t have a built-in feature to parse URLs. However, we can use the requests
library in Python to retrieve content from a URL.
from xhtml2pdf import pisaimport requests
def url_to_pdf(url, pdf_path): # Retrieve the HTML content from the URL response = requests.get(url) if response.status_code != 200: print(f"Failed to retrieve from URL: {url}") return False
html_content = response.text
# Create PDF with open(pdf_path, "wb") as pdf_file: pisa_status = pisa.CreatePDF(html_content, dest=pdf_file) return not pisa_status.err
# URL to retrieveurl_to_retrieve = "https://bbc.com"
# PDF path to savepdf_path = "bbc.pdf"
# Create PDFurl_to_pdf(url_to_retrieve, pdf_path)
In the url_to_pdf method of the code, the following steps are performed:
- We use the
requests
library to fetch the webpage content from the specified URL. - After receiving the content, we extract the text portion using
response.text
. - Next, for creating the PDF, we use pisa.CreatePDF. Here, we pass our HTML content and the name for the output PDF file.
Step 3: Create a PDF from custom HTML content using xhtml2pdf:**
from xhtml2pdf import pisa
def convert_html_to_pdf(html_string, pdf_path): with open(pdf_path, "wb") as pdf_file: pisa_status = pisa.CreatePDF(html_string, dest=pdf_file) return not pisa_status.err
# HTML contenthtml = '''<html> <head> <title>PDF Example</title> </head>
<body> <h1>Hey, this will turn into a PDF!</h1> </body></html>'''
# Create PDFpdf_path = "example.pdf"convert_html_to_pdf(html, pdf_path):
Creating a PDF from custom HTML content is similar to the process for URLs, with just one key difference: instead of passing a URL, we directly provide the actual HTML content to our creation method. The method then uses this custom HTML content to create the PDF.
Comparing Pyppeteer, python-pdfkit and xhtml2pdf
While each of these tools primarily focuses on converting HTML to PDF, they each offer unique features and methods.
Below, you’ll find a detailed table comparing these tools to help you select the one that best fits your requirements.
Library | Pros | Cons | Best For |
---|---|---|---|
Pyppeteer | Provides a full browser environment with comprehensive JS and CSS support. | Performance may vary. Requires Chrome/Chromium installation. | Handling intricate web content, single-page applications (SPAs), and dynamic JS content. |
xhtml2pdf | Pure Python easy setup. Good for simpler HTML/CSS documents without JavaScript. | Limited JavaScript and CSS support. | Simple HTML/CSS to PDF conversion tasks where JavaScript rendering is not needed. |
python-pdfkit | Fast native conversion. Good CSS support via wkhtmltopdf. | Limited JavaScript support. Requires wkhtmltopdf installation. | Various HTML to PDF tasks requiring good CSS rendering but limited JavaScript execution. |
In summary, the choice between Pyppeteer, xhtml2pdf, and python-pdfkit depends on your project’s specific requirements.
Pyppeteer shines when dealing with dynamic content, thanks to its complete browser automation features. On the other hand, xhtml2pdf provides a simple Python-focused solution for basic conversions.
python-pdfkit, which builds upon wkhtmltopdf, sits as a flexible option in between. Developers should consider the features, setup intricacies, and performance of each library in relation to their project’s needs to make the right decision.
A better approach: HTML to PDF using Templated
The examples above demonstrate how to convert HTML to PDF and web pages to PDF using libraries. However, when it comes to tasks like generating PDFs using templates or keeping track of generated PDFs, additional steps are required.
For instance, to keep track of generated PDFs, you’ll need to develop your own system for tracking the files created. Similarly, if you want to use custom templates, such as those for invoice or certificates generation, you must create and manage those templates manually.
An alternative solution is to utilize Templated.io, an API-based platform designed for PDF and Image generation, which is ideal for handling such use cases. Their PDF generation API is powered by a Chromium-based rendering engine that fully supports JavaScript, CSS, and HTML.
This approach simplifies the process and eliminates the need for extensive manual template management and tracking. To get started with PDF generation using Templated.io, follow the documentation and harness the power of this efficient solution.
1. Create PDFs with templates
Templated.io allows you to design and manage your templates.
After logging in you will see your Dashboard where you can manage your templated or create new ones:
From your Dashboard, you can design your own templates or customize a existing one from our Template Gallery. Bellow is the Certificate of Achievement Template you can use.
There are 100+ free templates available that you can pick and customize to your needs.
To start using Templated.io API, you need to get your API key that can be found on the API Integration tab on your dashboard.
Now that you have your Templated account ready, let’s see how you can integrate your application with the API. In this example we will be using a certificate template to generate PDFs.
import requestsimport json
# Initialize HTTP clientclient = requests.Session()
# API URLurl = "https://api.templated.io/v1/render"
# Set headersheaders = { "Content-Type": "application/json", "Authorization": "Bearer `${YOUR_API_KEY}`",}
# Payload datapayload = { "template": template_id, "format": "pdf", "modifications": [ { "layer": "date", "text": "02/10/2024" } { "layer": "name", "text": "John Doe" } { "layer": "signature", "text": "Dr. Mark Brown" } { "layer": "details", "text": "This certificate is awarded to John Doe in recognition of their successful " + "completion of Computer Sciente Degree on 02/10/2024." } ],}
# Serialize payload to JSONjson = json.dumps(payload)
# Make the POST requestresponse = client.post(url, data=json, headers=headers)
# Read the responseresponse = response.text
# Print the responseprint(response)
and If we check response
we have the following
{ "renderUrl":"PDF_URL", "status":"success", "template_id":"YOUR_TEMPLATE_ID"}
In the code above, using Templated to convert HTML to PDF is pretty simple. No additional libraries need to be installed. You only need to make a single API call, providing your data as the request body. That’s all there is to it!
You can use the renderUrl
from the response to download or distribute the generated PDF.
Other languages
You you want to learn how to convert HTML to PDF in other languages here are other resources for you to explore:
- How To Convert HTML to PDF with Java
- How To Convert HTML to PDF with C#
- How To Convert HTML to PDF with PHP
- How To Convert HTML to PDF with Node.js
Conclusion
PDF generation is now a standard part of every business application, and it shouldn’t be a source of stress for developers.
We’ve explored how to use third-party libraries for straightforward PDF generation. However, for more complex scenarios like template management, Templated offers a seamless solution through simple API calls.
To get started, sign up for a free account and begin automating your PDFs today!