· tutorials · 2 min read
How to Convert HTML to PDF with python-pdfkit
Uncover the straightforward approach to converting HTML to PDF in Python using the python-pdfkit library, accompanied by practical code examples.
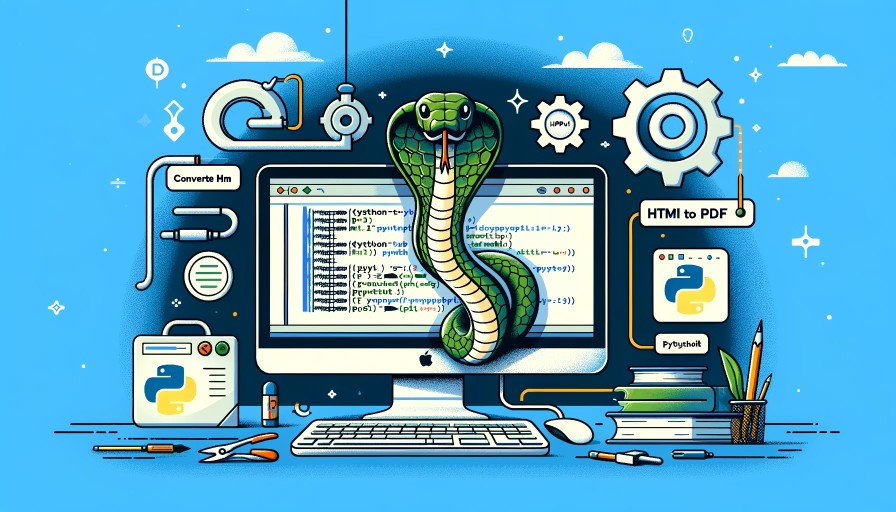
Introduction
Python-pdfkit is a Python wrapper for the wkhtmltopdf command-line tool, which converts HTML to PDF using WebKit. It’s a powerful tool for Python developers who need to generate PDFs from HTML content. This guide will walk you through converting HTML to PDF using python-pdfkit, with easy-to-understand code examples.
Why Python-pdfkit?
- Simplicity: Python-pdfkit provides a simple Pythonic interface to wkhtmltopdf, making PDF generation straightforward.
- Flexibility: It supports various customization options through wkhtmltopdf, such as page size, orientation, and margins.
- Quality Rendering: Leveraging WebKit, it renders HTML content with high fidelity, including styles and media.
Getting Started with Python-pdfkit
Step 1: Install Python-pdfkit and wkhtmltopdf
Before using python-pdfkit, you must install both the library and the wkhtmltopdf binary:
Install python-pdfkit using pip:
Terminal window pip install pdfkitInstall wkhtmltopdf:
- For Windows and macOS, download the appropriate installer from the wkhtmltopdf website.
- For Linux, you might be able to install it via your package manager. For example, on Ubuntu:
Terminal window sudo apt-get install wkhtmltopdf
Step 2: Convert HTML to PDF
With python-pdfkit, you can convert HTML strings, files, or URLs directly to PDF. Here’s a basic example of converting an HTML string to a PDF:
import pdfkit
html_content = """<!DOCTYPE html><html><head> <title>PDF Generation with Python-pdfkit</title></head><body> <h1>Python-pdfkit Demo</h1> <p>This is a simple demonstration of converting HTML to PDF in Python.</p></body></html>"""
# Convert HTML to PDFpdfkit.from_string(html_content, 'output.pdf')
print("PDF created successfully.")
In this example, pdfkit.from_string
takes the HTML content and the desired output PDF file name, generating a PDF document from the provided HTML.
Converting HTML Files and URLs to PDF
Python-pdfkit also allows you to convert HTML files or web pages directly to PDF:
From File:
pdfkit.from_file('input.html', 'output.pdf')From URL:
pdfkit.from_url('http://example.com', 'output.pdf')
Customization Options
You can customize the PDF output using options supported by wkhtmltopdf. For instance:
options = { 'page-size': 'Letter', 'margin-top': '0.75in', 'margin-right': '0.75in', 'margin-bottom': '0.75in', 'margin-left': '0.75in', 'encoding': "UTF-8", 'no-outline': None}
pdfkit.from_file('input.html', 'output.pdf', options=options)
Other Python libraries
There are other Python libraries capable of converting HTML to PDF and you can find more information about it in this article on How To Convert HTML to PDF with Python.
Conclusion
Python-pdfkit offers a straightforward and flexible way to convert HTML to PDF in Python applications, making it an excellent tool for generating reports, invoices, and other documents from web content. Its reliance on wkhtmltopdf ensures high-quality rendering, and its Pythonic interface simplifies the PDF generation process.