· tutorials · 5 min read
How To Convert HTML to PDF with Kotlin
Dive deep into the efficient methodologies for converting HTML to PDF in Kotlin, ensuring your project leverages the best available tools.
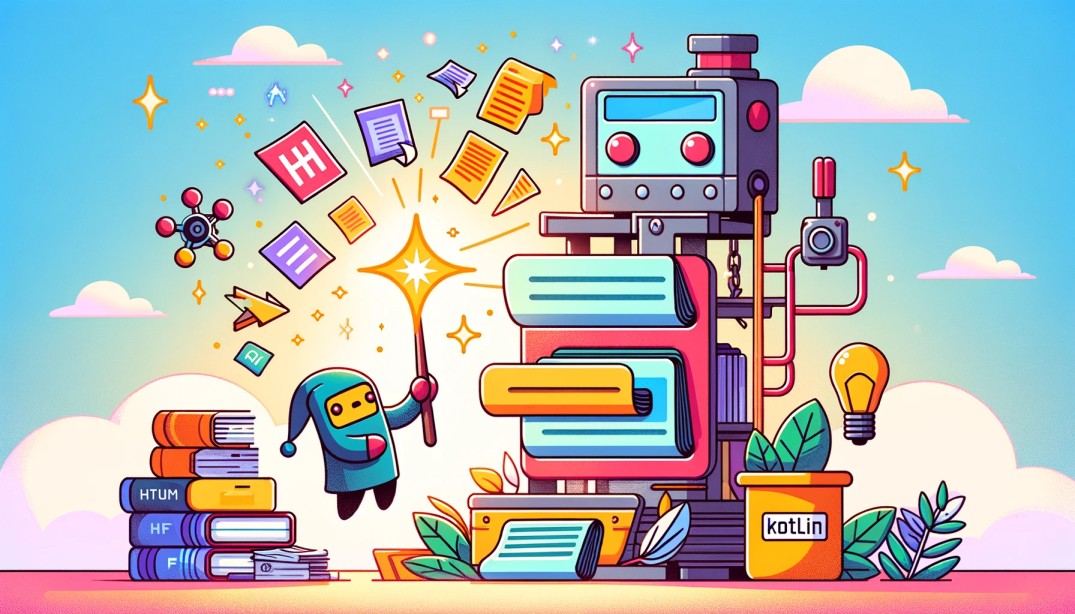
Introduction
In the digital age, the need to convert dynamic HTML content into a static PDF format is common across various applications, including generating reports, invoices, and other document types. Kotlin, with its concise syntax and interoperability with Java, offers a modern approach to tackle this task. This guide delves into several efficient techniques and tools for converting HTML to PDF, specifically tailored for Kotlin developers.
Why Choose HTML to PDF Conversion?
The decision to convert HTML to PDF is driven by several compelling reasons:
- Universality of HTML: HTML stands as the cornerstone of web content, offering a wide familiarity among developers. Utilizing HTML as a starting point for PDF generation taps into this universal knowledge base.
- Robust Support Network: The longevity and prevalence of HTML have fostered a comprehensive support network, ensuring developers have access to extensive resources and community expertise.
- Diverse Toolkit: The landscape of HTML to PDF conversion tools is rich and varied, providing options that cater to different needs, from simple document conversions to complex, design-rich publications.
- CSS for Customization: CSS empowers developers to style PDFs with the same degree of customization and precision as web pages, ensuring the final documents meet exact design specifications.
- Media-Rich Content: HTML’s capability to embed multimedia elements means that converted PDFs can retain a dynamic quality, incorporating images, hyperlinks, and more.
HTML to PDF using Kotlin Libraries
1. OpenPDF
OpenPDF, a successor to iText, is a notable open-source library that specializes in creating and manipulating PDF content. It provides a straightforward API for converting HTML to PDF in a Kotlin environment.
Step 1: Add OpenPDF Dependency
Incorporate OpenPDF into your Kotlin project by adding the dependency to your pom.xml
:
<dependency> <groupId>com.github.librepdf</groupId> <artifactId>openpdf</artifactId> <version>1.3.26</version></dependency>
Step 2: Kotlin Code to Convert HTML to PDF
A simple Kotlin example to demonstrate HTML to PDF conversion using OpenPDF:
import com.lowagie.text.Documentimport com.lowagie.text.Paragraphimport com.lowagie.text.html.simpleparser.HTMLWorkerimport com.lowagie.text.pdf.PdfWriterimport java.io.FileOutputStreamimport java.io.StringReader
fun main() { Document().apply { PdfWriter.getInstance(this, FileOutputStream("HtmlToPdf.pdf")) open() HTMLWorker(this).parse(StringReader("<html><body>This is my Project</body></html>")) close() } println("PDF created successfully.")}
2. Flying Saucer
Flying Saucer excels in rendering XHTML/CSS content to PDF. It’s an excellent choice for projects requiring precise layout and styling fidelity.
Step 1: Add Flying Saucer Dependency
To use Flying Saucer, add these dependencies:
<dependency> <groupId>org.xhtmlrenderer</groupId> <artifactId>flying-saucer-core</artifactId> <version>9.1.22</version></dependency><dependency> <groupId>org.xhtmlrenderer</groupId> <artifactId>flying-saucer-pdf</artifactId> <version>9.1.22</version></dependency>
Step 2: Kotlin Code to Convert HTML to PDF
The following Kotlin code snippet uses Flying Saucer to render an XHTML file to PDF:
import org.xhtmlrenderer.pdf.ITextRendererimport java.io.FileOutputStreamimport java.io.File
fun main() { ITextRenderer().apply { setDocument(File("input.html")) layout() createPDF(FileOutputStream("output.pdf")) } println("PDF created successfully with Flying Saucer.")}
3. iText
iText is a versatile library that not only facilitates HTML to PDF conversions but also offers extensive PDF manipulation capabilities.
Step 1: Add iText Dependency
Include iText by adding it to your pom.xml
:
<dependency> <groupId>com.itextpdf</groupId> <artifactId>html2pdf</artifactId> <version>3.0.1</version></dependency>
Step 2: Kotlin Code to Convert HTML to PDF
This example demonstrates converting HTML to PDF with iText:
import com.itextpdf.html2pdf.HtmlConverterimport java.io.FileInputStreamimport java.io.FileOutputStream
fun main() { HtmlConverter.convertToPdf(FileInputStream("input.html"), FileOutputStream("output.pdf")) println("PDF generated successfully with iText.")}
Leveraging Templated for Advanced HTML to PDF Conversion
While the libraries above offer great solutions for HTML to PDF conversion, managing complex templates or producing documents at scale can be challenging. Templated provides a powerful and easy-to-use API for generating PDFs from HTML templates, ideal for automating document generation processes in Kotlin applications.
This approach simplifies the process and eliminates the need for extensive manual template management and tracking. To get started with PDF generation using Templated, follow the documentation and harness the power of this efficient solution.
Using Templated API with Kotlin
Setting Up Templated API
First, obtain your API key from Templated and include the Templated API dependency in your project:
// Define your Templated API Keyval apiKey = "YOUR_API_KEY_HERE"
Kotlin Example to Generate PDF with Templated
The following Kotlin snippet demonstrates generating a PDF using Templated’s API:
import java.net.HttpURLConnectionimport java.net.URL
fun main() { val apiUrl = "https://api.templated.io/v1/render" val apiKey = "YOUR_API_KEY_HERE" val jsonInputString = """ { "template": "YOUR_TEMPLATE_ID", "layers": { "key": "value" } } """.trimIndent()
with(URL(apiUrl).openConnection() as HttpURLConnection) { requestMethod = "POST" setRequestProperty("Content-Type", "application/json; utf-8") setRequestProperty("Accept", "application/json") setRequestProperty("Authorization", "Bearer $apiKey") doOutput = true outputStream.use { os -> os.write(jsonInputString.toByteArray()) }
println("Response Code: $responseCode") inputStream.bufferedReader().use { reader -> println("Response: ${reader.readText()}") } }}
This code sends a POST request to Templated’s API, specifying the template and data for PDF generation. Replace "YOUR_TEMPLATE_ID"
with the actual ID of your template in Templated.
From your Dashboard, you can design your own templates or customize a existing one from our Template Gallery. Bellow is the Certificate of Achievement Template you can use.
There are 100+ free templates available that you can pick and customize to your needs.
Other languages
You you want to learn how to convert HTML to PDF in other languages here are other resources for you to explore:
- How To Convert HTML to PDF with Python
- How To Convert HTML to PDF with C#
- How To Convert HTML to PDF with PHP
- How To Convert HTML to PDF with Node.js
Conclusion
The shift towards digital document management underscores the necessity of efficient HTML to PDF conversion tools. Kotlin developers have a range of libraries at their disposal, each offering unique benefits. For complex or high-volume document generation needs, Templated’s API presents a streamlined, powerful solution.
By leveraging the right tools and approaches, developers can simplify the document generation process, enhance productivity, and focus on creating value in their applications.
Remember to explore Templated’s comprehensive documentation to fully utilize its capabilities for your document generation needs.
To get started, sign up for a free account and begin automating your PDFs today!