· tutorials · 5 min read
How to Convert HTML to PDF using Flying Saucer
Step into the world of Java-based HTML to PDF conversions with Flying Saucer, complete with easy-to-follow code examples.
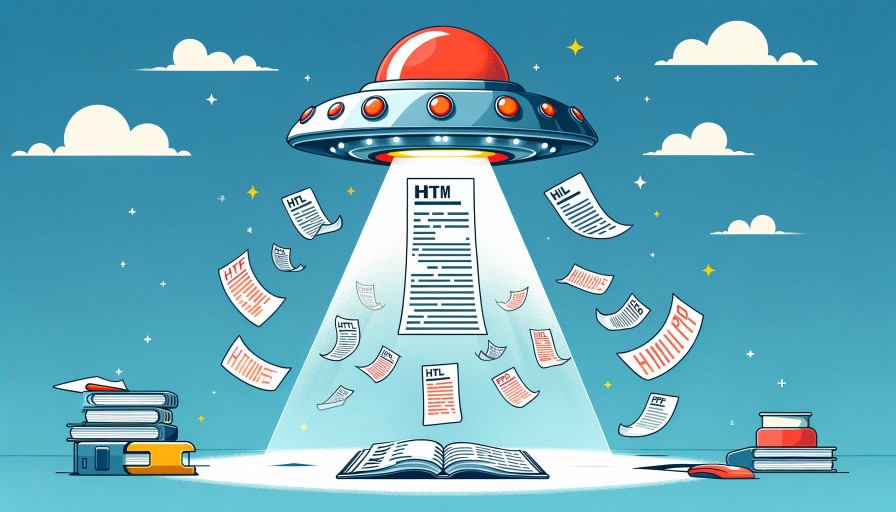
Introduction
Flying Saucer is a Java library that excels at converting XHTML/CSS content into PDFs, offering a high level of precision in rendering. This guide delves into the process of converting HTML to PDF using Flying Saucer, highlighting its ease of use and effectiveness with practical code examples.
Why Flying Saucer?
- CSS Support: Exceptional support for CSS 2.1, enabling sophisticated document styling.
- Ease of Use: Simple API for generating PDFs from HTML content.
- Integration: Seamless integration with Java applications and libraries.
Getting Started with Flying Saucer
Step 1: Add Flying Saucer Dependencies
To utilize Flying Saucer, you need to include its core library along with a PDF renderer, such as iText, in your project. For Maven users, add the following dependencies to your pom.xml
:
<dependency> <groupId>org.xhtmlrenderer</groupId> <artifactId>flying-saucer-core</artifactId> <version>9.1.22</version></dependency><dependency> <groupId>org.xhtmlrenderer</groupId> <artifactId>flying-saucer-pdf</artifactId> <version>9.1.22</version></dependency>
Step 2: Convert HTML to PDF with Flying Saucer
Flying Saucer requires well-formed XHTML to produce PDFs. If your HTML is not XHTML-compliant, you might need to preprocess it to conform to XHTML standards.
Here’s how you can convert XHTML to PDF using Flying Saucer:
import org.xhtmlrenderer.pdf.ITextRenderer;import java.io.FileOutputStream;import java.io.OutputStream;import com.lowagie.text.DocumentException;
public class HtmlToPdfFlyingSaucer { public static void main(String[] args) { String inputFile = "path/to/your/input.xhtml"; String outputFile = "path/to/your/output.pdf";
try { OutputStream os = new FileOutputStream(outputFile); ITextRenderer renderer = new ITextRenderer(); renderer.setDocument(new File(inputFile)); renderer.layout(); renderer.createPDF(os); os.close(); System.out.println("PDF created successfully."); } catch (DocumentException | IOException e) { e.printStackTrace(); } }}
In this example, ITextRenderer
is used to render the XHTML content as a PDF. The setDocument
method loads the XHTML file, layout
prepares the content for rendering, and createPDF
generates the PDF.
Enhancing PDFs with CSS
Flying Saucer’s strength lies in its CSS rendering capabilities. To leverage CSS for styling your PDF, include a <link>
to your CSS file within the <head>
of your XHTML document, just like in a regular HTML file.
A better approach: HTML to PDF using Templated
The examples above demonstrate how to convert HTML to PDF and web pages to PDF using libraries. However, when it comes to tasks like generating PDFs using templates or keeping track of generated PDFs, additional steps are required.
For instance, to keep track of generated PDFs, you’ll need to develop your own system for tracking the files created. Similarly, if you want to use custom templates, such as those for invoice or certificates generation, you must create and manage those templates manually.
An alternative solution is to utilize Templated, an API-based platform designed for PDF and Image generation, which is ideal for handling such use cases. Their PDF generation API is powered by a Chromium-based rendering engine that fully supports JavaScript, CSS, and HTML.
This approach simplifies the process and eliminates the need for extensive manual template management and tracking. To get started with PDF generation using Templated, follow the documentation and harness the power of this efficient solution.
Create PDFs with templates
Using Templated you can design your PDF template using a drag-and-drop editor.
Check the video below to see an example of the editor:
After logging in you will see your Dashboard where you can manage your templated or create new ones:
From your Dashboard, you can design your own templates or customize a existing one from our Template Gallery. Bellow is the Certificate of Achievement Template you can use.
There are 100+ free templates available that you can pick and customize to your needs.
To start using Templated API, you need to get your API key that can be found on the API Integration tab on your dashboard.
Now that you have your Templated account ready, let’s see how you can integrate your application with the API. In this example we will be using a certificate template to generate PDFs.
import java.net.HttpURLConnection;import java.net.URL;import java.io.OutputStream;import java.io.BufferedReader;import java.io.InputStreamReader;import org.json.JSONObject;
public class TemplatedApiRequest { public static void main(String[] args) { try { URL url = new URL("https://api.templated.io/v1/render"); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("POST"); conn.setRequestProperty("Content-Type", "application/json"); conn.setRequestProperty("Authorization", "Bearer ${YOUR_API_KEY}"); conn.setDoOutput(true);
JSONObject payload = new JSONObject(); payload.put("template", "template_id"); payload.put("format", "pdf");
JSONObject layers = new JSONObject();
// Add layers to the changes object changes.put("date", "02/10/2024"); changes.put("name", "John Doe"); changes.put("signature", "Dr. Mark Brown"); changes.put("details", "This certificate is awarded to John Doe in recognition of their successful completion of Computer Science Degree on 02/10/2024.");
payload.put("changes", changes);
// Add layers to the changes object JSONObject dateLayer = new JSONObject(); dateLayer.put("text", "02/10/2024"); layers.put("date", dateLayer);
JSONObject nameLayer = new JSONObject(); nameLayer.put("text", "John Doe"); layers.put("name", nameLayer);
JSONObject signatureLayer = new JSONObject(); signatureLayer.put("text", "Dr. Mark Brown"); layers.put("signature", signatureLayer);
JSONObject detailsLayer = new JSONObject(); detailsLayer.put("text", "This certificate is awarded to John Doe in recognition of their successful completion of Computer Science Degree on 02/10/2024."); layers.put("details", detailsLayer);
payload.put("layers", layers);
OutputStream os = conn.getOutputStream(); os.write(payload.toString().getBytes()); os.flush(); os.close();
int responseCode = conn.getResponseCode(); System.out.println("Response Code : " + responseCode);
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream())); String inputLine; StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close();
// Print the response System.out.println(response.toString()); } catch (Exception e) { e.printStackTrace(); } }}
and If we check response
we have the following
{ "renderUrl":"PDF_URL", "status":"success", "template":"YOUR_TEMPLATE_ID"}
In the code above, using Templated to convert HTML to PDF is pretty simple. No additional libraries need to be installed. You only need to make a single API call, providing your data as the request body. That’s all there is to it!
You can use the renderUrl
from the response to download or distribute the generated PDF.
Other Java libraries
There are other Java libraries capable of converting HTML to PDF and you can find more information about it in this article on How To Convert HTML to PDF with Java.
Conclusion
Flying Saucer offers a Java-based solution for generating PDFs from HTML content, particularly excelling in rendering CSS-styled documents. Its straightforward API and powerful CSS rendering capabilities make it an ideal choice for applications requiring precise control over document layout and style in their PDF conversions.